Reverse Engineering Mobile Apps for Oracle EBS – part 1
Published on: Author: Richard Velden Category: OracleIn this article we will show the inner workings of the new mobile apps for Oracle E-Business Suite (EBS). We use Oracle’s own Timecard app as an example.
In my previous blog we have shown where to download the Oracle Timecard app for customization. Apart from customizing these apps we would also like to create our own mobile app using Oracle Mobile Application Framework (MAF). So before simply proceeding with developing our own mobile app for Oracle E-Business Suite, we thought it would be wise to first check Oracle’s own implementation in detail.
Inner Workings
In this blog we will focus on the inner workings of Oracle’s Timecard app. We will do this by focusing on one particular user action: the flow from pressing the “Save Time Entry” button, all the way to Oracle EBS. We start off with opening the Timecard MAA in JDeveloper.
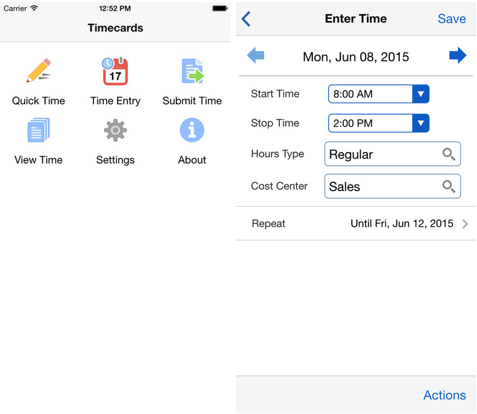
Timecard app structure
In JDeveloper we see 2 projects:
- ApplicationController
- ViewController
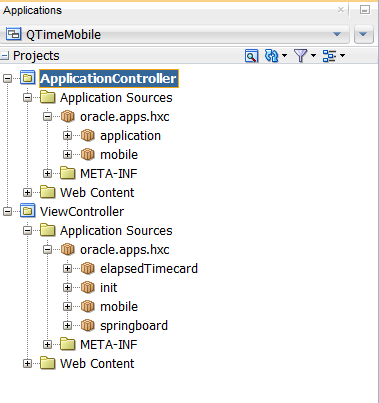
Each project has a specific use. In this app we only see two projects. Another structure one could apply is an application with one ApplicationController project, and multiple ViewController projects.
ApplicationController
The ApplicationController project contains application-wide functionality. Here we can find skins, translation bundles, application images and splash screens.
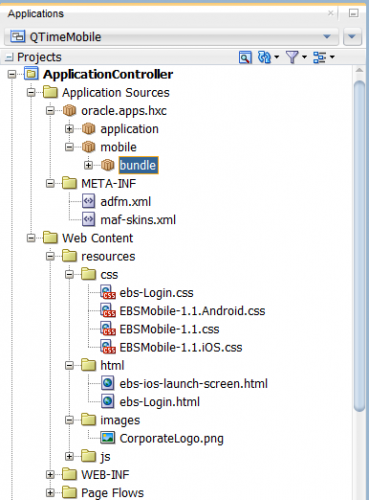
Also login, security, and the main navigation flow of the app is defined in this project by means of the maf-application.xml.
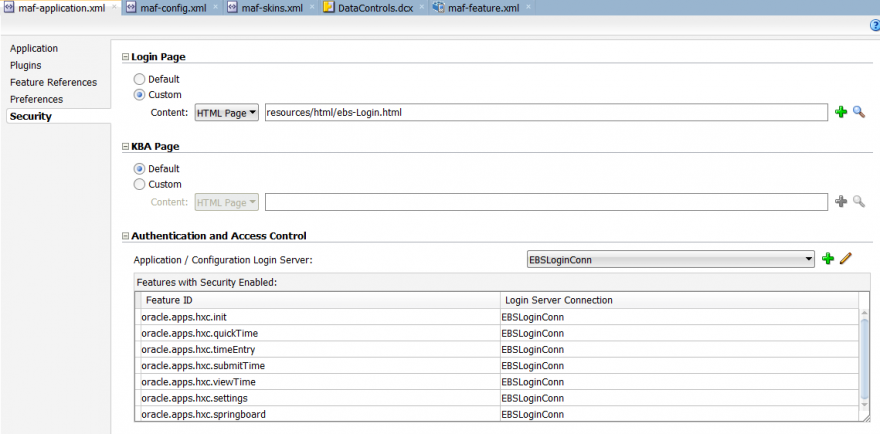
The ApplicationController project is essentially a consumer of the ViewController project. It defines which application features to show where on the Navigation Bar or Springboard (see figures 5 and 6).
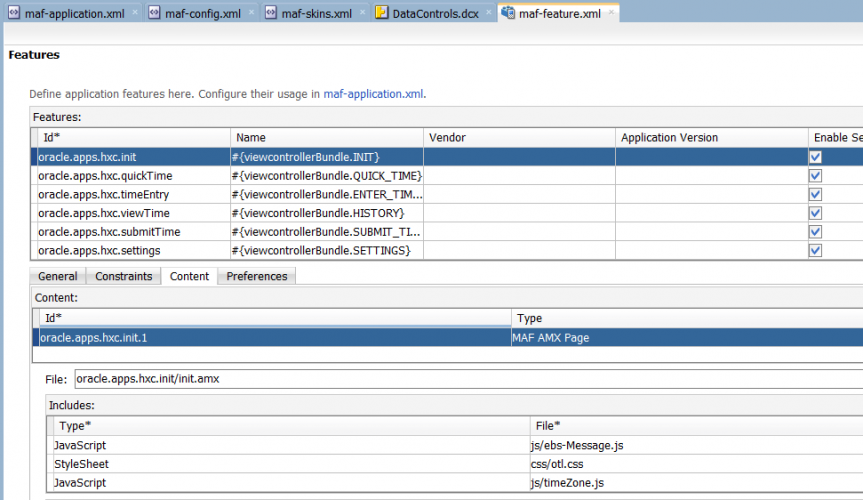
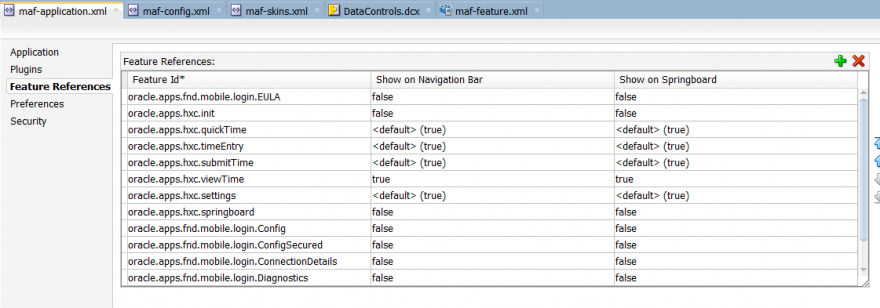
In the Timecard app the maf-application.xml specifies to use a custom springboard (see figure 7). Instead of using the framework springboard, a ‘feature’ defined in the ViewController project is used. This features is implemented by an AMX-page.
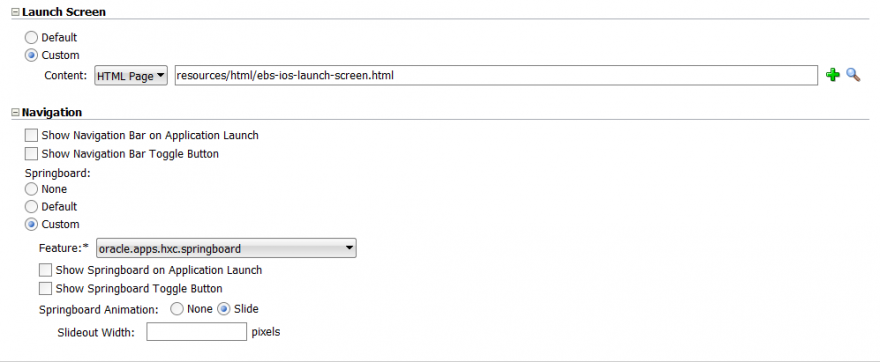
ViewController
In MAF applications the ViewController project contains all the UI components (.amx files) and controller logic (TaskFlows, Java Beans). ViewController projects list all their available features in a file called maf-features.xml. In figure 8 we have selected the ‘feature’ oracle.apps.hxc.timeEntry which is implemented by the TE_TF TaskFlow. In figure 9 the TimeEntry TaskFlow diagram is shown.
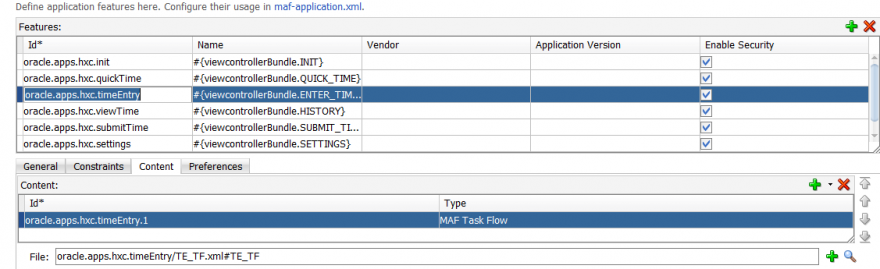
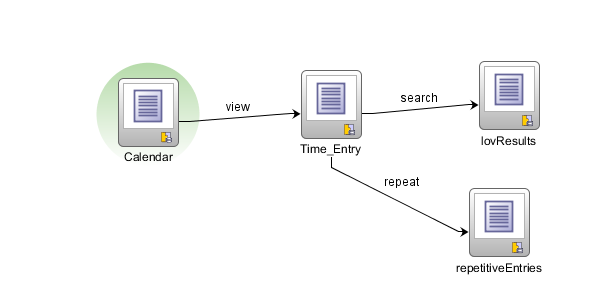
The Calendar view will enable us to select a date, once selected the Time Entry view is opened for entering time for that date. Once time period and attributes (project, task, etc.) have been entered, the save button can be pressed. This button is bound to a particular DataControl (see figure 10, 11, 12 and 13).

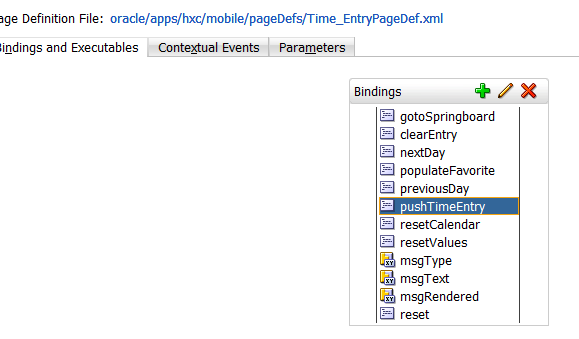
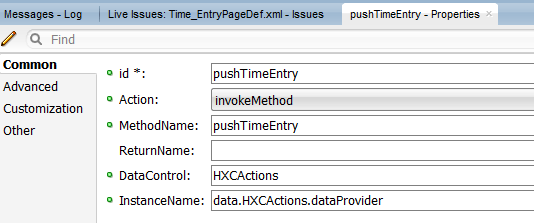
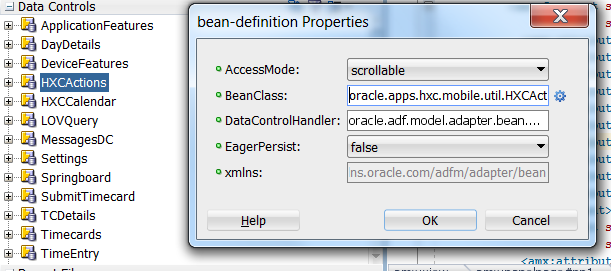
When checking the implementation of JavaBean HXCActions we only find a JDeveloper generated stub. This stub only contains some method names, and not the implementation details. If you need to know the actual implementation we need to decompile these classes.
Libraries
The remaining logic of the Timecard app is contained in java libraries. See figure 14 how to show these libraries in the applications view.
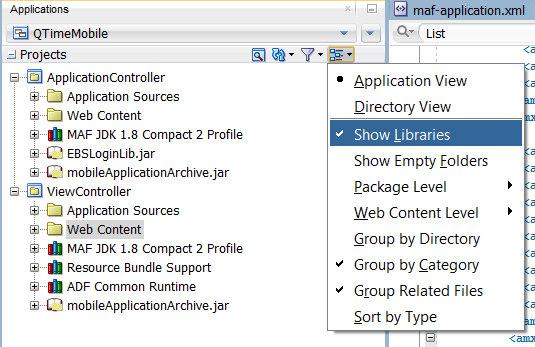
EBSLoginLib.jar contains login related helper classes, specifically for E-Business Suite. It contains helpers for connecting to EBS login REST services, as well as a responsibility picker component.
The other library, mobileApplicationArchive.jar contains more application related functionality. Here we find the HXCActions.class or one of the Webservice client classes responsible for calling EBS webservices (see figure 15).
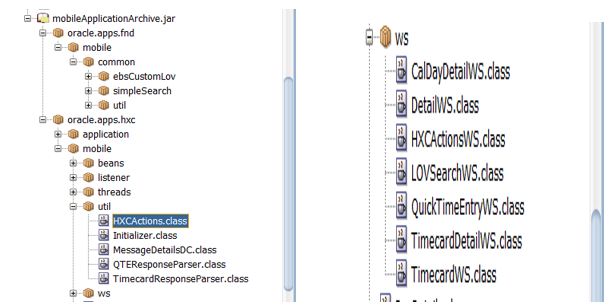
Decompiling libraries
To actually see the implementation of these libraries we need to decompile the Java classes. Unzip the mobileApplicationArchive.jar file which can be found in folder ViewController\classlib. Decompile all Java classes using jad.
How? Navigate to folder: "mobileApplicationArchive" and open the command prompt here. One can recursively invoke jad and decompile all classes in the proper directory structure using: jad -d . -s java -r **/*.class
Now we are ready to investigate the inner workings of this TimeEntry DataControl encountered earlier. We open the Java source file used by this DataControl: oracle.apps.hxc.mobile.util.HXCActions
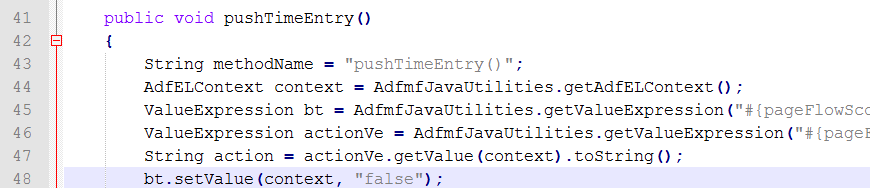
By investigating these sources we noticed that view input is stored (and retrieved) using pageFlow variables.
String st = getSpacedOutput("#{pageFlowScope.startTime}");
st = getSpacedOutput("#{pageFlowScope.stopTime}");
Later on we found the actual REST service call going to Oracle EBS (see figure 17). The input string of this call (String xml) contains values such as startTime as shown above.
throws Exception { RestUtil rest = new RestUtil(); rest.setConnection("EBSConn"); rest.setRequestType("POST"); rest.setRequestURL("/OA_HTML/RF.jsp?function_id=HXC_MOB_PUSH_DETAILS"); rest.setRequestXML(xml); try { response = rest.processRequest(); } { AppLogger.logException(cl, methodName, e); throw e; } return response; }
Please note that a RestUtil class is used to call a REST endpoint on the EBS server at: http://ebsurl:port/OA_HTML/RF.jsp?function_id=HXC_MOB_PUSH_DETAILS
Mobile REST Service Implementation in Oracle EBS
Just to dig a little deeper, we would like to check the REST service implementation on the Oracle EBS side. Earlier we already noticed the absence of pre-deployed REST services in the Integrated SOA Gateway. Back then we wondered where to find the implementation of the REST services used by the mobile apps.
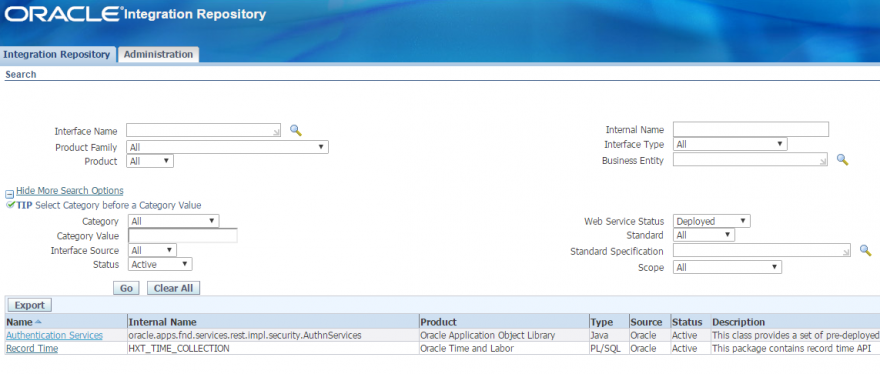
Although we always did find the Authentication Services, there were no other APIs active and deployed.
So where is my service? Luckily, the function_id, found in the Java source code in the previous section, did ring a bell.
/OA_HTML/RF.jsp?function_id=HXC_MOB_PUSH_DETAILS
We navigate to the System Administrator responsibility in Oracle EBS and select Application -> Function. Now search for the function HXC_MOB_PUSH_DETAILS.
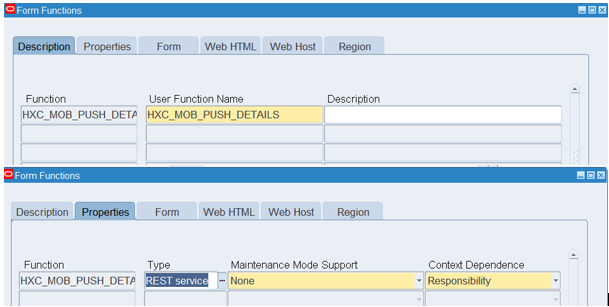
In figure 19 we show two of the search tabs available for this function. It shows us a pointer to a REST service implementation. When investigating the other tabs we find the following:
- In the Web HTML we find HTML Call: OAR
- In the Form tab we find a form parameter: type=model&amName=oracle.apps.hxc.mbl.server.OTLMobileAM&methodName=pushDetails
Using this OAR HTML call, Oracle Development enabled a REST interface for the ‘pushDetails’ method. A method which has been especially designed for their OTL Mobile app. Let’s be honest, the module name “OTLMobileAM” kind of implies that it has been created for this mobile app. A method, probably subject to change, and should thus not be used as an API by other developers!
This kind of shows us why we should not bluntly copy one of the Oracle mobile apps, and use that as a starting point for our own. No-one will ensure this ‘internal API’ will stay the same in the next patch. Basically this recommendation can also be found in the “Oracle EBS Mobile Developer Guide” [1]. But it is good to see these reasons in detail as well.
In figure 20 a small subset when one searches for all similar REST functions.
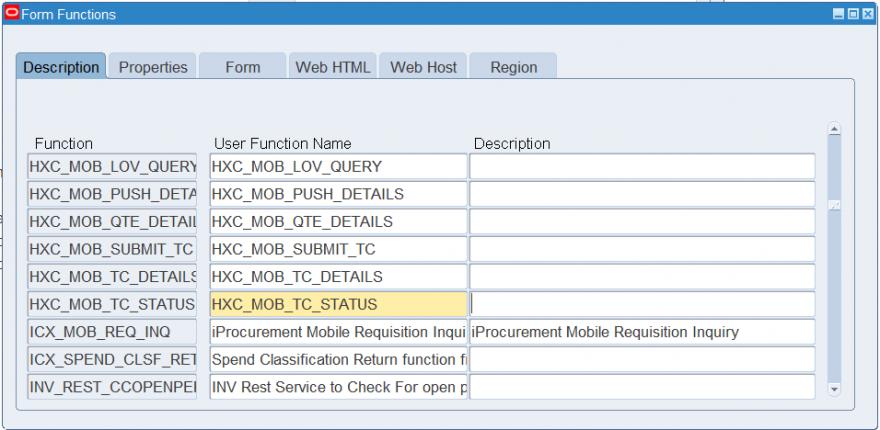
Follow up
In this first part of our ‘reverse engineering’ article we have briefly discussed both the ADF structure of the app, as well as the service implementation in Oracle EBS. In the second part of this article we will focus on the authentication services used by EBS mobile apps.
References
[1] Oracle® E-Business Suite Mobile Foundation Developer's Guide
[3] Introduction to Oracle Mobile Application Framework
[4] Using Mobile Application Archives for Enterprise Distribution
Great!!
Helpful information! Reverse engineering of already developed mobile <a href="https://yuktisolutions.com/blog/best-mobile-app-development-company-in-delhi">app</a>.
Link fail.