Custom XPath functions in Service Bus
Gepubliceerd: Auteur: Milco Numan Categorie: OracleHow can you create custom XPath functions in Oracle Service Bus 12c? And how can you use these in both XSLT and XQuery? Let’s find it out. I like to show you how they’re different in behaviour.
The context of my post comes from one of my projects, where we were migrating quite some (stateless) services from the SOA (BPEL) platform to Service Bus. Since our clients were already ‘virtualized’ to our clients (i.e. clients invoked them through the Service Bus), we could easily change the implementation platform without changing the service contracts.
For the transformation, we were reusing the existing XSL transformations, so a couple of the custom XPath functions had to be made available in Service Bus. Additionally, we were also introducing the DVM (Domain Value Maps) as a replacement for a custom coded lookup-implementation, created when DVMs did not yet exist. For this purpose, we had to create a custom XPath wrapper function, in order to implement some custom logging that the customer did not want to lose.
Test XPath function
As a simple scenario, I am using base-64 encoding and decoding to be implemented as custom XPath functions (code is shared through GitHub). In order to test the custom XPath function, I have created some very simple proxies and pipelines that do not route to any other service but instead just call upon a transformation in order to test my custom functions:
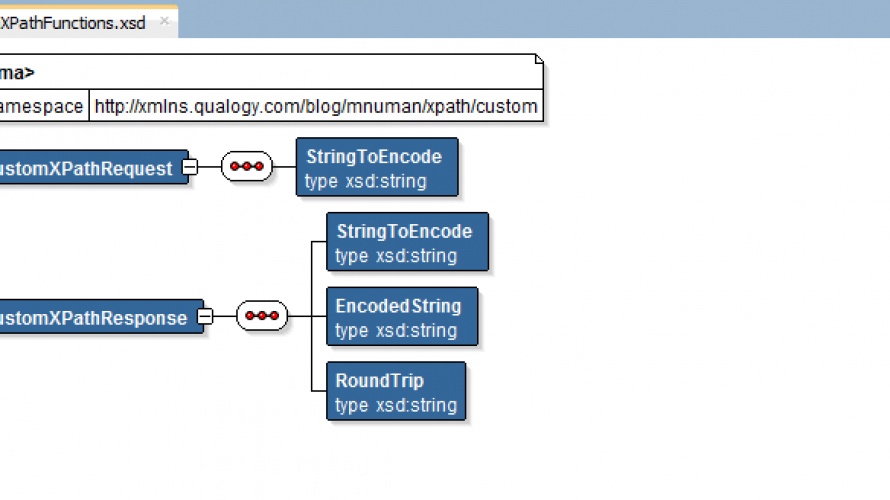
Child elements
In the reply element I have three different child elements, the first contains the untransformed contents of the input string and the second contains the base64 encoded contents. The last element contains the value after invoking the decode operation on the encoded string, to verify that the inverse operation restores the original value.
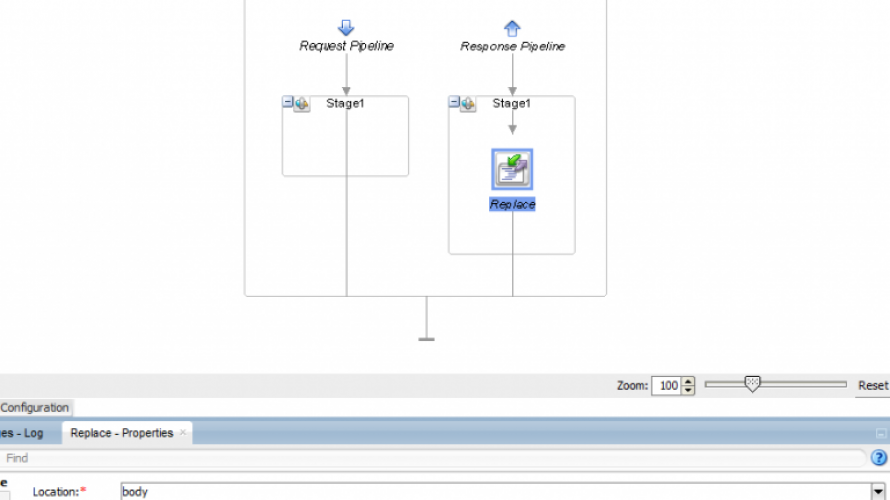
package com.qualogy.soa.xpath; import oracle.soa.common.util.Base64Decoder; import oracle.soa.common.util.Base64Encoder; import javax.xml.xpath.XPathFunctionException; public class Base64 { /** * Apply base64 encoding. * @param input * @return input after encoding into Base64 * @throws XPathFunctionException on failure */ public static String encodeBase64(final String input) throws XPathFunctionException{ String enc = null; try { enc = Base64Encoder.encode(input); } catch(Exception exc) { } return enc; } /** * Apply base64 decoding. * @param input * @return input after decoding from Base64 * @throws XPathFunctionException on failure */ public static String decodeBase64(final String input) throws XPathFunctionException{ String dec = null; try { dec = Base64Decoder.decode(input); } catch(Exception exc) { } return dec; }
Implementation in Java
The custom XPath function is implemented in Java, as a static method call. The logic itself is already implemented in one of the underlying JARs from the platform, so I am basically wrapping this for both Base-64 encode and decode operations. The only dependency that needs to be resolved is to the oracle.soa.common.util.Base64Decoder & Base64Encoder, by adding the fabric-runtime.jar from your FMW_HOME/soa/soa/modules/oracle.soa.fabric_11.1.1/ to the classpath.
package com.qualogy.soa.xpath; import oracle.soa.common.util.Base64Decoder; import oracle.soa.common.util.Base64Encoder; import javax.xml.xpath.XPathFunctionException; public class Base64 { /** * Apply base64 encoding. * @param input * @return input after encoding into Base64 * @throws XPathFunctionException on failure */ public static String encodeBase64(final String input) throws XPathFunctionException{ String enc = null; try { enc = Base64Encoder.encode(input); } catch(Exception exc) { } return enc; } /** * Apply base64 decoding. * @param input * @return input after decoding from Base64 * @throws XPathFunctionException on failure */ public static String decodeBase64(final String input) throws XPathFunctionException{ String dec = null; try { dec = Base64Decoder.decode(input); } catch(Exception exc) { } return dec; }
Usage of custom XPath functions in XSLT
After compiling this code into a JAR file, you’re good to go as far as XSLT is concerned. The only thing that is required is to put the JAR somewhere in the classpath where the Service Bus can access it (of course, you will need to restart the Service Bus after you placed the jar…). For example:
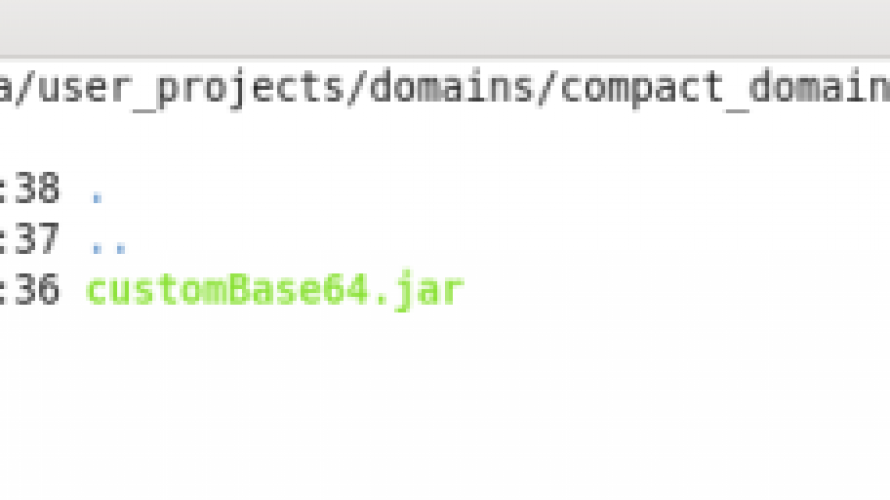
After making the JAR available (preferably in $DOMAIN_HOME/config/osb/xpath-functions), you can access the custom XPath functions it implements. You should:
- define a namespace in your XSL transformation that maps to http://www.oracle.com/XSL/Transform/java/com.qualogy.soa.xpath.Base64. The first part is fixed, the second part is the fully qualified classname that implements your custom XPath function
- invoke the custom XPath function by using a namespace-qualified function name. The namespace is defined in the step above, the function name is the exact same function name as it is being called in the implementation (so that would be encodeBase64 or decodeBase64).
Below the implementation of the XSL transformation (some of the clutter introduced by the JDeveloper mapper has been removed):
<xsl:stylesheet version="1.0" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:ns0="http://xmlns.qualogy.com/blog/mnuman/xpath/custom" xmlns:xsl="http://www.w3.org/1999/XSL/Transform"> <xsl:template match="/"> <ns0:CustomXPathResponse> <ns0:StringToEncode> <xsl:value-of select="/ns0:CustomXPathRequest/ns0:StringToEncode"/> </ns0:StringToEncode> <ns0:EncodedString> <xsl:value-of select="customxp:encodeBase64(/ns0:CustomXPathRequest/ns0:StringToEncode)"/> </ns0:EncodedString> <ns0:RoundTrip> <xsl:value-of select="customxp:decodeBase64(customxp:encodeBase64(/ns0:CustomXPathRequest/ns0:StringToEncode))"/> </ns0:RoundTrip> </ns0:CustomXPathResponse> </xsl:template> </xsl:stylesheet>
Proof of the pudding
As always, the proof of the pudding is in the ‘testing’. This can be easily verified after deploying your service using the Service Bus Console’s tester for the proxy service:
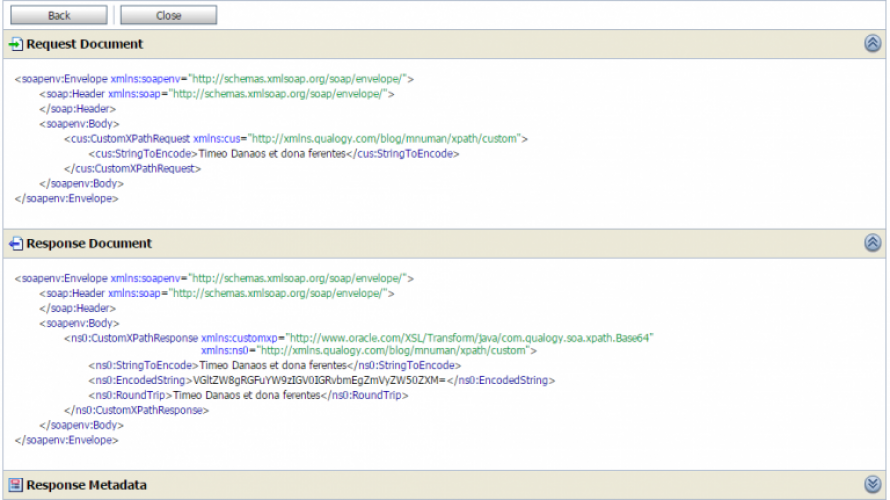
Usage of custom XPath functions in XQuery
In order to use custom XPath function in XQuery, some additional steps have to be performed, apart from making the JAR file available in Service Bus’ Classpath.
First, you must create a mapping file, mapping the namespace qualified function name that you want to use in XQuery to its Java implementation:
<?xml version="1.0" encoding="UTF-8" ?> <xpf:xpathFunctions xmlns:xpf="http://www.bea.com/wli/sb/xpath/config"> <xpf:category id="Qualogy Custom Functions"> <xpf:function> <xpf:name>base64encode</xpf:name> <xpf:comment>Perform Base-64 encoding on the input string, e.g. base64encode($input as xs:string) as xs:string</xpf:comment> <xpf:namespaceURI>http://www.qualogy.com/soa/xpath</xpf:namespaceURI> <xpf:className>com.qualogy.soa.xpath.Base64</xpf:className> <xpf:method>java.lang.String encodeBase64(java.lang.String)</xpf:method> <xpf:isDeterministic>false</xpf:isDeterministic> <xpf:scope>Pipeline</xpf:scope> <xpf:scope>SplitJoin</xpf:scope> </xpf:function> <xpf:function> <xpf:name>base64decode</xpf:name> <xpf:comment>Perform Base-64 decoding on the input string, e.g. base64decode($input as xs:string) as xs:string</xpf:comment> <xpf:namespaceURI>http://www.qualogy.com/soa/xpath</xpf:namespaceURI> <xpf:className>com.qualogy.soa.xpath.Base64</xpf:className> <xpf:method>java.lang.String decodeBase64(java.lang.String)</xpf:method> <xpf:isDeterministic>false</xpf:isDeterministic> <xpf:scope>Pipeline</xpf:scope> <xpf:scope>SplitJoin</xpf:scope> </xpf:function> </xpf:category> </xpf:xpathFunctions>
Mapped XQuery function
So in this example, we have mapped an XQuery function {http://www.qualogy.com/soa/xpath}base64encode to the java method encodeBase64 implemented in com.qualogy.soa.xpath.Base64. Note that you also need to specify the method signature (input/output arguments, whether it is deterministic — it actually is and the allowed use of the function: Pipeline and/or SplitJoin).
Next, you need to make this mapping file available inside your Service Bus runtime environment. This file will be placed alongside the implementation jar. There are no naming restrictions, it should only be a valid XML file.
After restarting the Service Bus, the custom XPath function may also be used from XQuery… or can it?
XQuery Design Time
After defining the custom XPath function to the runtime, we should have been able to use it in an XQuery, but alas: JDeveloper will prevent this since we are yet to make this function available to the design time environment as well:
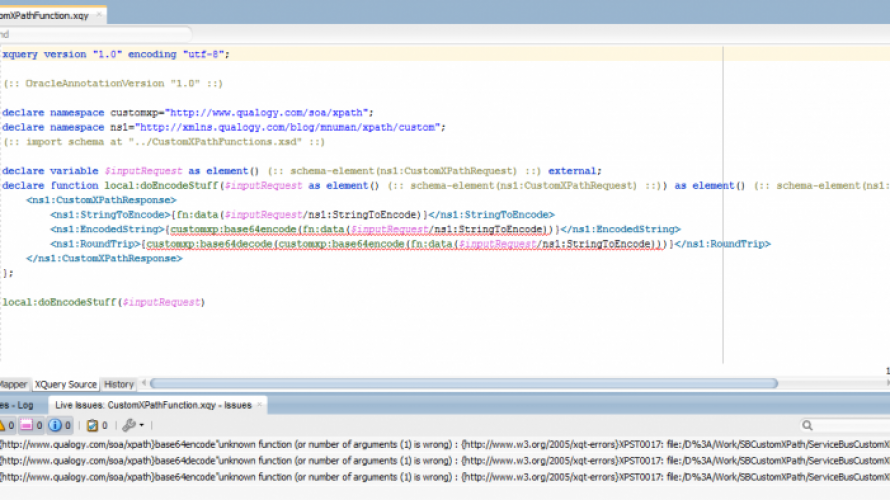
As you can see, JDeveloper fails to recognize the new XPath function. We should have made this available to JDeveloper as well, by placing both the JAR and the mapping file in the PRODUCT_HOME/osb/config/xpath-functions for JDeveloper:
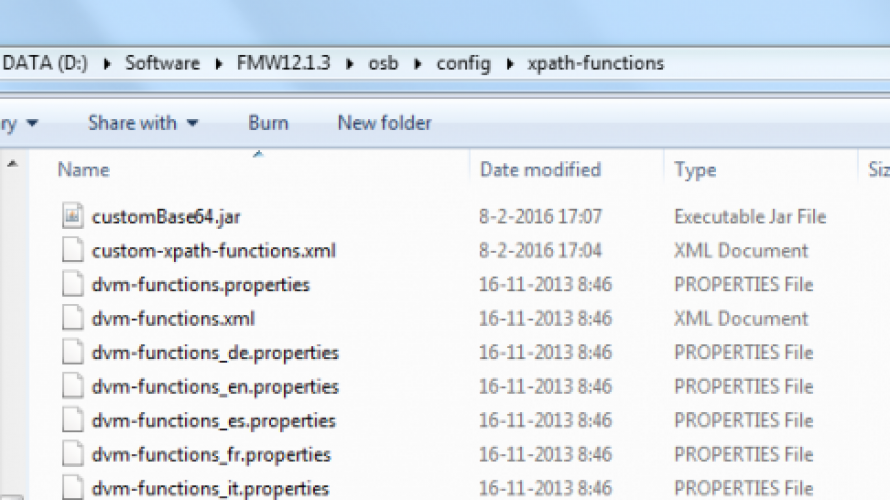
xquery version "1.0" encoding "utf-8"; (:: OracleAnnotationVersion "1.0" ::) declare namespace customxp="http://www.qualogy.com/soa/xpath"; declare namespace ns1="http://xmlns.qualogy.com/blog/mnuman/xpath/custom"; (:: import schema at "../CustomXPathFunctions.xsd" ::) declare variable $inputRequest as element() (:: schema-element(ns1:CustomXPathRequest) ::) external; declare function local:doEncodeStuff($inputRequest as element() (:: schema-element(ns1:CustomXPathRequest) ::)) as element() (:: schema-element(ns1:CustomXPathResponse) ::) { <ns1:CustomXPathResponse> <ns1:StringToEncode>{fn:data($inputRequest/ns1:StringToEncode)}</ns1:StringToEncode> <ns1:EncodedString>{customxp:base64encode(fn:data($inputRequest/ns1:StringToEncode))}</ns1:EncodedString> <ns1:RoundTrip>{customxp:base64decode(customxp:base64encode(fn:data($inputRequest/ns1:StringToEncode)))}</ns1:RoundTrip> </ns1:CustomXPathResponse> }; local:doEncodeStuff($inputRequest)
For XQuery then, the actual invocation just uses the defined namespace qualified name from the mapping file. Of course, the service yields the same results for a happy flow.
Handling failures
What is most interesting, is the different behaviour in case of failures for both transformation engines. In this project, we were surprised a couple of times on different environments that had been configured manually… almost a guarantee for differences. I won’t go into the details, but in more than one occasion, either the JAR file or the mapping file were missing or a wrong version had been made available.
To simulate this behaviour in my ‘Jip-and-Janneke’ (for non-Dutch: simple) scenarios, I moved the JAR and XML file out of the way and restarted the Service Bus. When the actual implementation is unavailable, the outputs for the unhappy flows are differing like night and day:
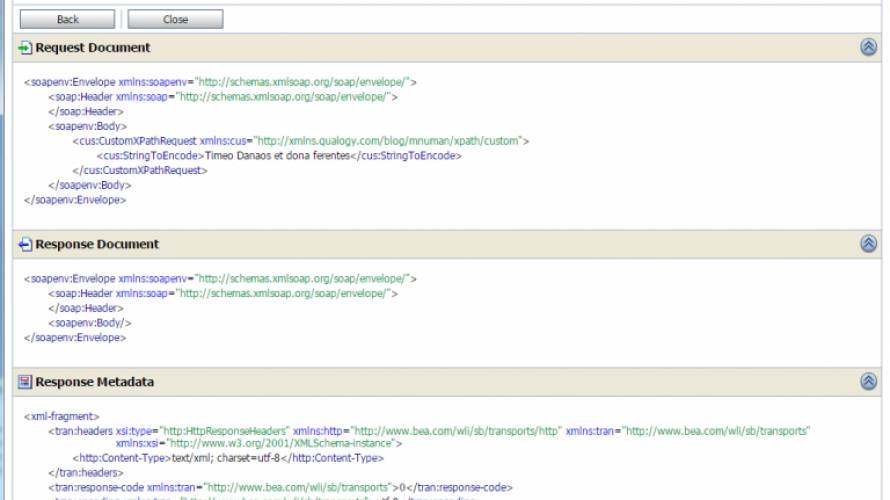
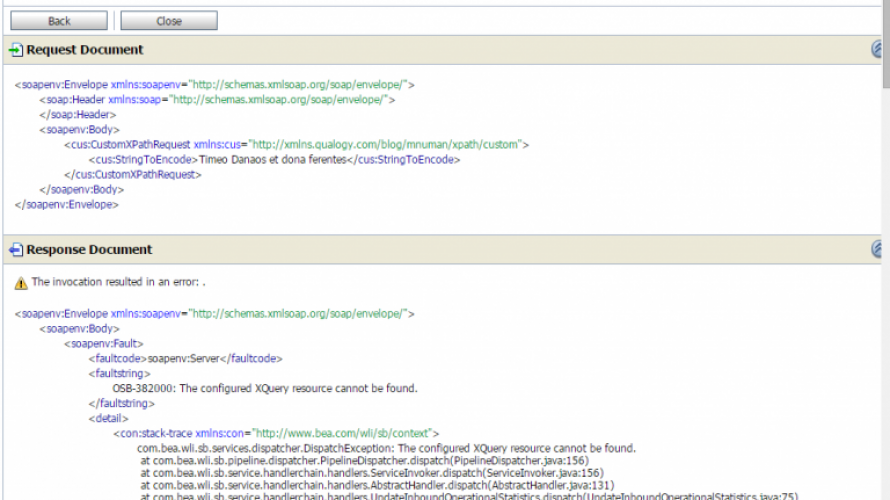
XSLT unhappy flow
As you can see from the XSLT unhappy flow, it simply returns an empty response. But with a regular ‘OK’ status (HTTP 200), whereas the XQuery unhappy flow will return a proper SOAP Fault message showing it could not find the actual custom transformation function.
The XSLT implementation does output some information to the console:
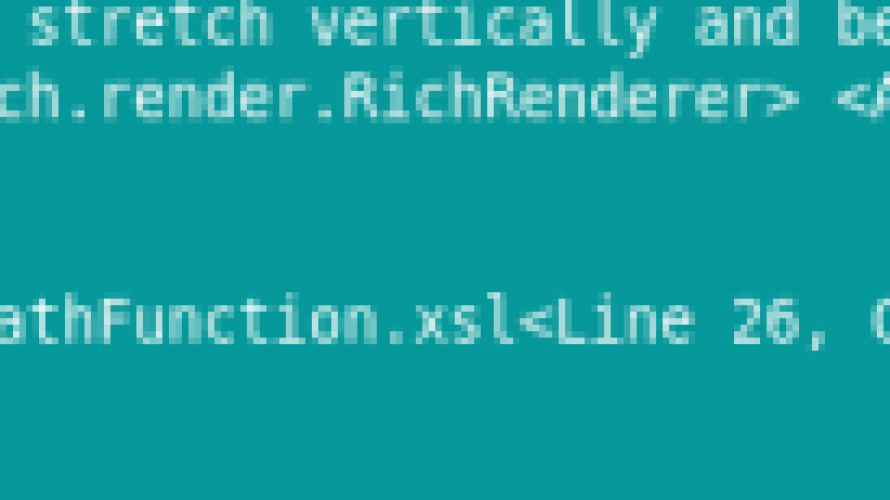
Always inspect the gifts
So at the end of the day you can use your custom XPath function implementations in both XSTL and XQuery. Whatever transformation language you choose to use will depend on things like possibility of reuse of existing resources, knowledge and preference of the developer. Perhaps it is also prescribed by your customer’s or project’s development standards. However, you should be aware that although usage XPath functions in XQuery require some more steps. This also comes with the advantage of clearer error messages. So you may fear the Greeks, but you should always inspect the gifts (see *explanation)!
*Explanation
The quote used for testing “Timeo Danaos et dona ferentes” is taken from the Virgil’s Aeneid and refers to the Trojan Horse.
How is it different from doing a java callout ?