Taking some REST (part 1)
Gepubliceerd: Auteur: Milco Numan Categorie: Oracle[Deze blog is alleen in het Engels beschikbaar]
In this blog post, I will provide a general introduction of REST, explain some of the choices made and lay some of the ground work. The second part of this blog series will discuss the token management part while the third and final part describes the actual creation of the SOAP services providing the proxy to the external REST APIs.
With the introduction of SOA Suite 12c, the Oracle JCA REST adapter was introduced for use in both SOA and Service Bus 12c. This enables SOA developers to expose REST interfaces to their service consumers. A study by the Oracle A-team shows that in certain scenarios response times on mobile platforms may be reduced by an order of magnitude by consuming REST services instead of their SOAP equivalents.
However, using the REST adapter you cannot only expose REST interfaces to your own service implementations (inbound REST), but you can also consume REST services (outbound REST). In this series of blogs, I will demonstrate how I implemented a use case of “outbound” API management for a proof of concept, where the REST services were exposed as SOAP web services to our internal clients (which are largely “REST unaware”). An additional dimension is provided by the fact that the REST APIs invoked are secured by OAuth 2.0, so also some token management is needed in order to successfully invoke the service.
What about Security?
I am glad you asked. As SOAP has quite a number of standardized extensions in the realms of orchestration and security, this is very much “terra incognita” in the REST world. Well, not really. As REST is leveraging the HTTP protocol as the transport mechanism, a first step in security would be to use HTTPS (HTTP over SSL) to prevent an intermediary from eavesdropping on the communication between the service and the client. However, this will only prevent the interception of messages, it does not provide the server with any method of determining the origin of the request. Using HTTP Basic Authentication may be an option to force the client to sent some identification to the server, but the problem is that this scenario is not very useful for application to application message exchange as it does not provide options to provide temporary access or to revoke the access.
Enter OAuth, “an open protocol to allow secure authorization in a simple and standard method from web, mobile and desktop applications.” Version 2.0 of this protocol has been published in 2012 and is widely in use for securing access and authorization to services, e.g. Google, LinkedIn, Facebook, Dropbox and Paypal to name a few.
What is REST?
What is REST? And how does if differ from SOAP? Well, first of all REST and SOAP are intended to access (remote) Web Services. Where SOAP is a heavy-weight protocol, involving predefined message structures and formats, REST is defined very loosely as an “architectural style”. Messages transmitted to SOAP web services are always encoded as XML data structures, whereas the payload in REST can be either XML or JSON. The latter seems to be the preferred format nowadays, I came across a site describing JSON as “The Fat-Free Alternative To XML“.
In REST, you are manipulating “resources” (types of objects, e.g. customers, orders, items and the like) using standard HTTP methods. You’d use the GET method on a resource to retrieve a single instance or collection, use the HTTP POST method to create a new one, update a resource instance using HTTP PUT and the HTTP DELETE takes care of removing an object.
If you want to learn more about REST, I specifically recommend the REST API Tutorial.
What about Security?
I am glad you asked. As SOAP has quite a number of standardized extensions in the realms of orchestration and security, this is very much “terra incognita” in the REST world. Well, not really. As REST is leveraging the HTTP protocol as the transport mechanism, a first step in security would be to use HTTPS (HTTP over SSL) to prevent an intermediary from eavesdropping on the communication between the service and the client. However, this will only prevent the interception of messages, it does not provide the server with any method of determining the origin of the request. Using HTTP Basic Authentication may be an option to force the client to sent some identification to the server, but the problem is that this scenario is not very useful for application to application message exchange as it does not provide options to provide temporary access or to revoke the access.
Enter OAuth, “an open protocol to allow secure authorization in a simple and standard method from web, mobile and desktop applications.” Version 2.0 of this protocol has been published in 2012 and is widely in use for securing access and authorization to services, e.g. Google, LinkedIn, Facebook, Dropbox and Paypal to name a few.
OAuth 2.0
For the proof of concept, I selected the Google Tasks API; this is a simple API, can be used at no charge and has different resources and styles of invocation and the Google documentation is quite extensive. This API is secured by Google’s Identity Service, using OAuth 2.0.
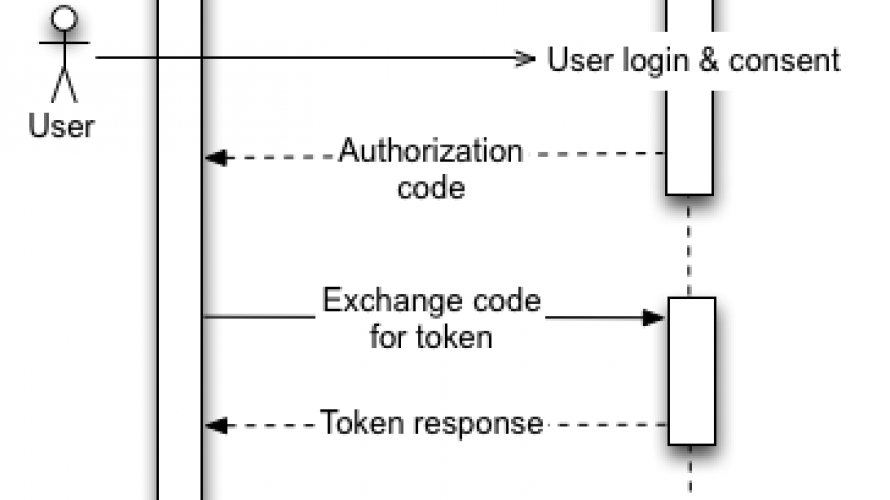
The above diagram describes a basic flow for obtaining and using OAuth 2.0:
- some (web) application requests access to a protected resource
- the user is presented with a form to login and consent in the requestor gaining access to the resource with the request scope
- upon approval, the Google Identity Service returns an authorization code to your application
- your application needs to redeem the authorization code into a token; your application will receive both an access token and a refresh token
- the access token may be used for calling the API(s) it has gained access to on behalf of the consenting user
- usually, access tokens have a limited lifespan, usually one hour; after the access token expires, the refresh token can be used to regain access
There is a lot more to OAuth than I present over here; access can be revoked and there exist multiple other access scenarios, the above being one of the most common.
Scenario
Since the POC only involved the service part, I have no web application to request the user’s consent and obtain an authorization token. Luckily, this part can also be performed by using a regular browser, where the authorization will be returned from the Google Identity Server to the browser. This token can then be input into a service call.
Since the service calls require (almost) no orchestration and are stateless, I will be implementing the flows in Oracle Service Bus 12c; access and refresh tokens will be cached in the embedded Coherence server running in WebLogic server (although a more robust scenario would be to store the temporary access token in-memory and store the refresh token persistently in the database).
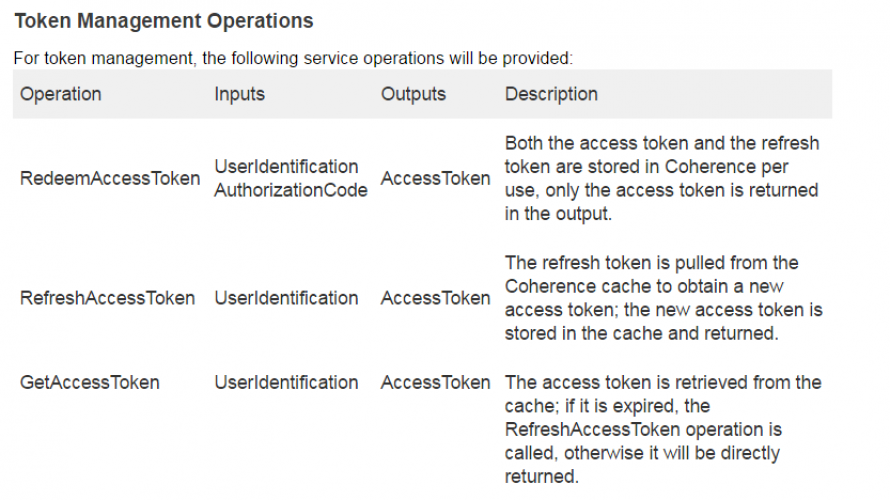
The UserIdentification consists of two parts, one identifying the token issuer (identity provider) and the other being the user’s email address.
The code is created in Oracle’s SOA 12.1.3 Virtual Box image, using the SOA Domain. This environment has a Coherence cache configured out of the box (adapter-local).
Setting Up – Google
For setting up the OAuth 2.0 client (my webservices) I first created a new user account on Google (q.oauth.demo@gmail.com) for hosting the “project”. Through Google’s Developers Console, you need to setup a project and create an OAuth 2.0 Client:
Obtaining an OAuth ClientId and Client Secret
In the process, you need to provide information to be shown on your consent screen and enable the Google APIs you will be invoking from your application:
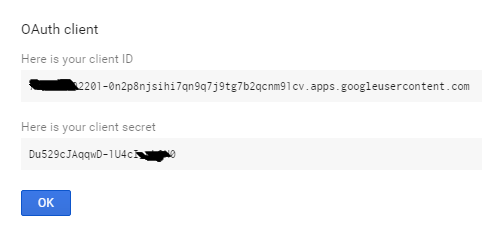
Obtaining an OAuth ClientId and Client Secret
In the process, you need to provide information to be shown on your consent screen and enable the Google APIs you will be invoking from your application:
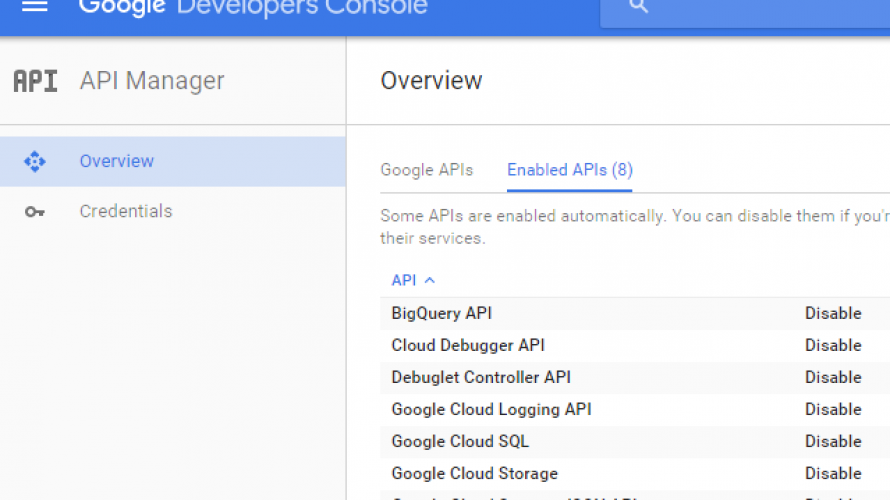
Now, with your user, project and OAuth identity setup, it is time to ask the user’s permission to use the Tasks API on his behalf. As explained, normally there would be a web application requesting this permission; for demonstration purposes, I will be conducting this approval step from the Web Browser. This can be accomplished by simply entering the following URL in the browser’s address bar:
https://accounts.google.com/o/oauth2/v2/auth?response_type=code&client_id=&redirect_uri=urn:ietf:wg:oauth:2.0:oob&scope=https://www.googleapis.com/auth/tasks
(The parameters are documented here, the scopes your are requesting access to depend on the APIs being called – look here for the task scopes). After logging into Google, the user is presented with the following page that informs him that his consent is required by Q-OAuth-Demo for maintaining his tasks:
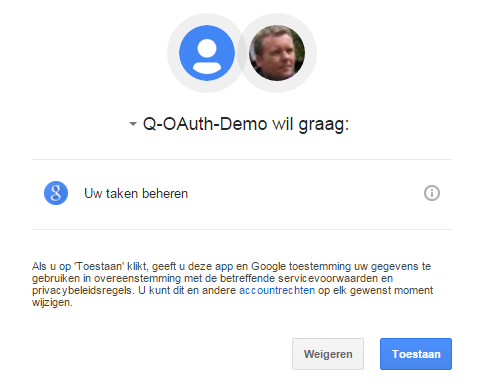
Consent screen for project request access
After approving the request, a one-time use authorization code is returned to the browser session, to be exchanged for a token pair:
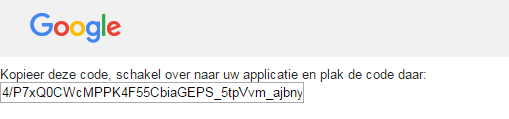
In the next part of this blog series, I will be describing the token management services in more detail that may be invoked after obtaining the authorization code.
Read more in part 2 of my blog.